ChatGPT를 활용한 뱀(스네이크) 게임 만들기
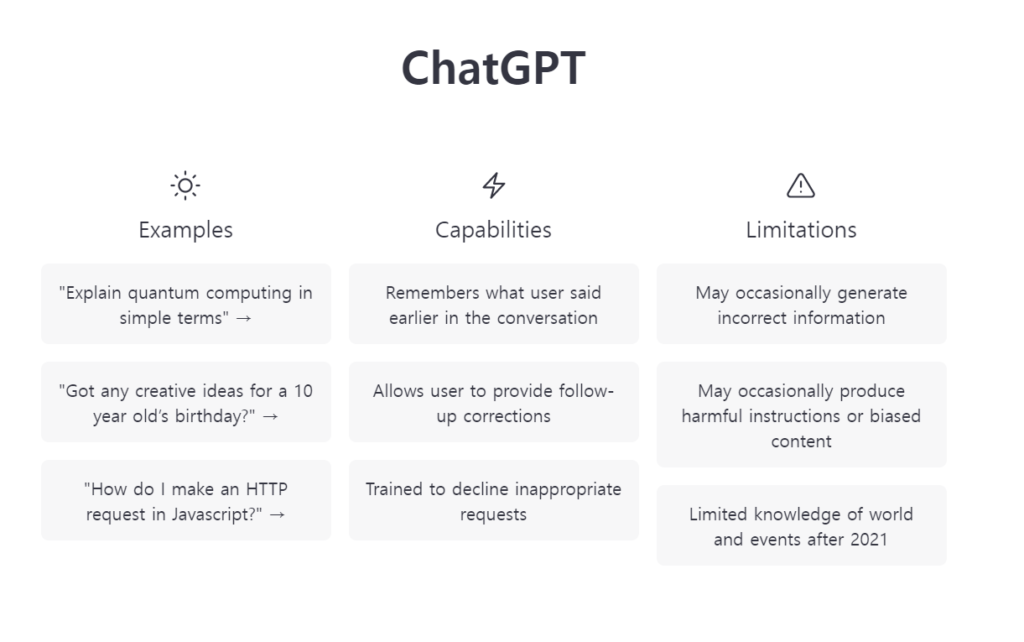
최근 화제가 되고 있는 생성형 AI ChatGPT를 활용하여 뱀 게임(??)을 만들어 보겠습니다.
ChatGPT의 경우 Python를 기반으로 하고 있어 대부분 Python 코드로 프로그래밍을 알려줍니다.
※ PC에 파이썬이 설치되어 있어야합니다.
나: Give me a python snake game |
ChatGPT: Sure! Here’s a simple implementation of the classic Snake game in Python using the Pygame library: |
ChatGPT
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 |
import pygame import time import random # Initialize Pygame pygame.init() # Set the size of the game window window_width = 500 window_height = 500 game_window = pygame.display.set_mode((window_width, window_height)) # Set the title of the game window pygame.display.set_caption('Snake Game') # Set the colors used in the game white = (255, 255, 255) black = (0, 0, 0) red = (255, 0, 0) # Set the font used in the game font = pygame.font.SysFont(None, 30) # Define the size of the snake block and the speed of the game block_size = 10 clock = pygame.time.Clock() fps = 15 # Define a function to display a message on the screen def message_to_screen(msg, color): screen_text = font.render(msg, True, color) game_window.blit(screen_text, [window_width/6, window_height/3]) # Define the main game loop def gameLoop(): game_exit = False game_over = False # Define the starting position of the snake and its initial length lead_x = window_width / 2 lead_y = window_height / 2 lead_x_change = 0 lead_y_change = 0 snake_list = [] snake_length = 1 # Define the starting position of the food food_x = round(random.randrange(0, window_width - block_size) / 10.0) * 10.0 food_y = round(random.randrange(0, window_height - block_size) / 10.0) * 10.0 # Game loop while not game_exit: while game_over == True: game_window.fill(white) message_to_screen("Game over! Press Q to quit or C to play again", red) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.KEYDOWN: if event.key == pygame.K_q: game_exit = True game_over = False elif event.key == pygame.K_c: gameLoop() # Event handling loop for event in pygame.event.get(): if event.type == pygame.QUIT: game_exit = True if event.type == pygame.KEYDOWN: if event.key == pygame.K_LEFT: lead_x_change = -block_size lead_y_change = 0 elif event.key == pygame.K_RIGHT: lead_x_change = block_size lead_y_change = 0 elif event.key == pygame.K_UP: lead_y_change = -block_size lead_x_change = 0 elif event.key == pygame.K_DOWN: lead_y_change = block_size lead_x_change = 0 # Check if the snake has hit the edges of the window if lead_x >= window_width or lead_x < 0 or lead_y >= window_height or lead_y < 0: game_over = True # Update the position of the snake's head lead_x += lead_x_change lead_y += lead_y_change # Draw the background and the food game_window.fill(white) pygame.draw.rect(game_window, red, [food_x, food_y, block_size, block_size]) # Add the snake's head to the beginning of the snake_list snake_head = [] snake_head.append(lead_x) |
ChatGPT 사용해보기 https://chat.openai.com